Algebra Fundamentals
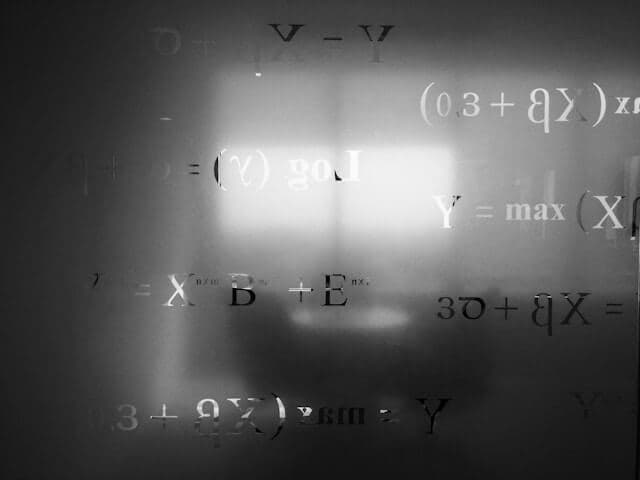
This is the first part of the series Mathematics for Machine Learning with Python.
Intro to equations
Starting with an equation: 2x + 3 = 9
to find the the x
. x = 3
x = -41
x + 16 == -25 # True
Working with fractions
x = 45
x / 3 + 1 == 16 # True
Variables in both sides
x = 1.5
3 * x + 2 == 5 * x -1 # True
Linear Equations
Creating a dataframe with the x
and y
columns and their values
import pandas as pd
from matplotlib import pyplot as plt
df = pd.DataFrame({'x': range(-10, 11)})
df['y'] = (3 * df['x'] - 4) / 2
Simple way to plot and show the graph
plt.plot(df.x, df.y, color="grey")
plt.xlabel('x')
plt.ylabel('y')
plt.grid()
plt.show()
Annotate the points when x = 0 and y = 0
plt.annotate('x-intercept', (1.333, 0))
plt.annotate('y-intercept', (0, -2))
plt.show()
Finding the slope through the equation:
slope = Δy/Δx
Slope is usually represented by the letter m
m = (y2 - y1) / (x2 - x1)
Getting these two points, we can infer the slope value: (0, -2), (6, 7)
m = (7 - (-2)) / (6 - 0)
m = 1.5
It means that when moving one unit to the right (x-axis), we need to move 1.5 units up (y-axis) to get back to the line.
m = 1.5
yInt = -2
mx = [0, 1]
my = [yInt, yInt + m]
Systems of Equations
In equations with two variables x
and y
, we can use elimination to find the values when the intersect with each other
x + y = 16
10x + 25y = 250
With elimination, you'll find out that x = 10
and y = 6
when the lines intersect.
x = 10
y = 6
print((x + y == 16) & ((10 * x) + (25 * y) == 250))
When plotting the lines of those equations, we get this graph
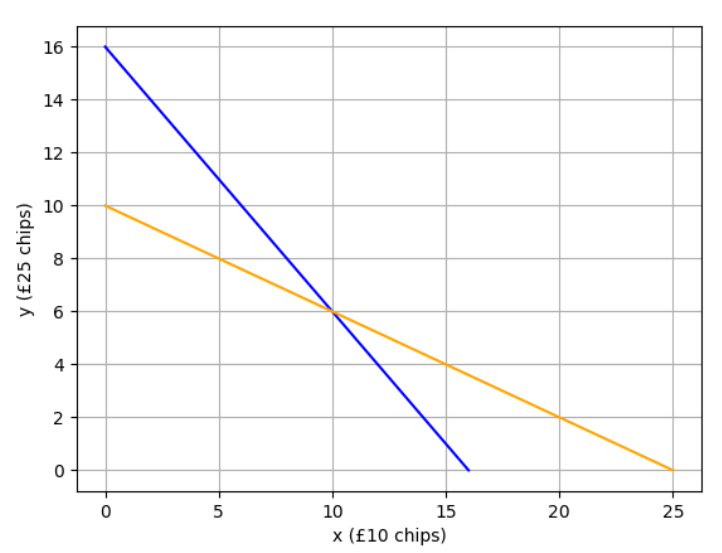
Here's how we generate the code
chipsAll10s = [16, 0]
chipsAll25s = [0, 16]
valueAll10s = [25, 0]
valueAll25s = [0, 10]
plt.plot(chipsAll10s, chipsAll25s, color='blue')
plt.plot(valueAll10s, valueAll25s, color="orange")
plt.xlabel('x (£10 chips)')
plt.ylabel('y (£25 chips)')
plt.grid()
plt.show()
Exponentials & Logarithms
Exponentials have a simple case that's squaring a number: 2² = 2 x 2 = 4
.
2 ** 2 # 4
Radicals (roots) is useful to calculate a solution for exponential
?² = 9
√9 = 3
∛64 = 4
In Python we can use math.sqrt
to get the square root of a number and a trick to get the cube root.
math.sqrt(25) # 5
round(64 ** (1. / 3)) # 64 ^ 1/3 = ∛64 = 4
To find the exponent for a given number and base, we use the logarithm
4 ^ ? = 16
log₄(16) = 2
The math
module has a log
function that receive the number and the base
math.log(16, 4) # 2.0
math.log(29) # 3.367295829986474
math.log10(100) # 2.0
Solving equations with exponentials:
2y = 2(x^4)((x^2 + 2x^2) / x^3)
2y = 2(x^4)(3x^2 / x^3)
2y = 2(x^4)(3x^-1)
2y = 6(x^3)
y = 3(x^3)
We can exemplify this with Python
df = pd.DataFrame ({'x': range(-10, 11)})
# add a y column by applying the slope-intercept equation to x
df['y'] = 3 * df['x'] ** 3 # this is the equation we simplified above
plt.plot(df.x, df.y, color="magenta")
plt.xlabel('x')
plt.ylabel('y')
plt.grid()
plt.axhline()
plt.axvline()
plt.show()
It generates this graph:
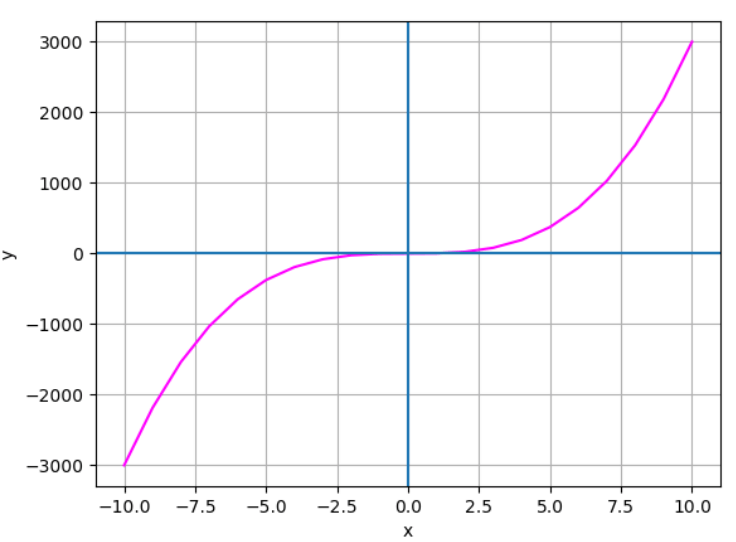
Polynomials
A polynomial is an algebraic expression containing one or more terms.
12x³ + 2x - 16
The terms themselves include:
- Two coefficients(12 and 2) and a constant (-16)
- A variable (x)
- An exponent (³)
Simplifying the polynomial:
x³ + 2x³ - 3x - x + 8 - 3
3x³ - 4x + 5
And we can compare both equations in Python
from random import randint
x = randint(1,100)
(x**3 + 2 * x**3 - 3 * x - x + 8 - 3) == (3 * x**3 - 4 * x + 5)
# True
Factorization
Factorization
is the process of restating an expression as the product of two expressions.
-6x²y³
You can get this value by performing the following multiplication:
(2xy²)(-3xy)
So, we can say that 2xy² and -3xy are both factors of -6x²y³.
from random import randint
x = randint(1,100)
y = randint(1,100)
(2 * x * y**2) * (-3 * x * y) == -6 * x**2 * y**3
The Greatest Common Factor
(GCF) is the highest value that is a multiple of both number n1
and number n2
.
We can apply this idea to polynomials too.
15x²y
9xy³
The GCF of these polynomial is 2xy
Factorization is useful for expressions like the differences of squares:
x² - 9
x² - 3²
(x - 3)(x + 3)
We generalize this idea to this expression: a² - b² = (a - b)(a + b)
Ensure this is true:
from random import randint
x = randint(1,100)
(x**2 - 9) == (x - 3) * (x + 3)
This is also true for perfect squares
x² 10x + 25 (x - 5)(x + 5) (x + 5)²
And we can generalize to this expression: (a + b)² = a² + 2ab + b²
Ensure this with Python
from random import randint
a = randint(1,100)
b = randint(1,100)
a**2 + b**2 + (2 * a * b) == (a + b)**2
Quadratic Equations
Use the complete the square method to solve quadratic equations. Take this following equation as an example:
x² + 24x + 12²
Can be factored to this:
(x + 12)²
OK, so how does this help us solve a quadratic equation? Well, let's look at an example:
y = x² + 6x - 7
Let's start as we've always done so far by restating the equation to solve x for a y value of 0:
x² + 6x - 7 = 0
Now we can move the constant term to the right by adding 7 to both sides:
x² + 6x = 7
OK, now let's look at the expression on the left: x² + 6x. We can't take the square root of this, but we can turn it into a trinomial that will factor into a perfect square by adding a squared constant. The question is, what should that constant be? Well, we know that we're looking for an expression like x² + 2cx + c², so our constant c is half of the coefficient we currently have for x. This is 6, making our constant 3, which when squared is 9 So we can create a trinomial expression that will easily factor to a perfect square by adding 9; giving us the expression x² + 6x + 9.
However, we can't just add something to one side without also adding it to the other, so our equation becomes:
x² + 6x + 9 = 16
So, how does that help? Well, we can now factor the trinomial expression as a perfect square binomial expression:
(x + 3)² = 16
And now, we can use the square root method to find x + 3:
x + 3 = √16
So, x + 3 is -4 or 4. We isolate x by subtracting 3 from both sides, so x is -7 or 1:
x = -7, 1
Functions
Functions are usually the same how it's in programming. Data in, data out.
f(x) = x² + 2
f(3) = 11
def f(x):
return x**2 + 2
f(3) # 11
Bounds of function: domain
Imagine a function g(x) = (12 / 2x)²
, where {x ∈ ℝ | x ≠ 0}
In Python:
def g(x):
if x != 0:
return (12 / 2 * x)**2
x = range(-100, 101)
y = [g(a) for a in x]
Conditional: for k(x)
{
0, if x = 0,
1, if x = 100
}
In Python:
def k(x):
if x == 0:
return 0
elif x == 100:
return 1
x = range(-100, 101)
y = [k(a) for a in x]